1. Introduction to Java
Java is a high-level, object-oriented, and platform-independent programming language. It was designed by James Gosling at Sun Microsystems (now Oracle) and released in 1995. Java is widely used for building applications in web development, mobile development (Android), enterprise software, and more.
Key features of Java include:
- Write Once, Run Anywhere (WORA): Java programs can run on any device with a Java Virtual Machine (JVM).
- Object-Oriented: Focuses on creating reusable code through objects and classes.
- Secure: Provides a secure runtime environment through features like bytecode verification.
- Robust: Exception handling and memory management make Java programs reliable.
- Multi-threaded: Java supports concurrent programming.
2. What are JRE, JDK, and JIT?
JRE (Java Runtime Environment):
- The JRE provides the environment to run Java programs.
- It includes the Java Virtual Machine (JVM) and the libraries required to execute Java applications.
- Developers and users who only want to run Java programs typically install the JRE.
JDK (Java Development Kit):
- The JDK is a complete development environment for creating Java programs.
- It includes:
- The JRE
- Development tools like the compiler (
javac
), debugger, and other utilities.
- Libraries and APIs required for Java development.
- To write and compile Java code, the JDK is essential.
JIT (Just-In-Time) Compiler:
- The JIT is a part of the JVM that optimizes Java bytecode execution.
- When a Java program runs, the JVM interprets bytecode. The JIT compiles frequently executed parts of the bytecode into native machine code for faster execution.
- This process happens at runtime, making Java programs more efficient without manual optimization.
Java achieves platform independence through the use of bytecode and the JVM:
- When a Java program is compiled, it is converted into an intermediate format called bytecode (.class files).
- Bytecode is not machine-specific; it is designed to run on the JVM.
- Each operating system has its own implementation of the JVM (e.g., Windows, Linux, macOS).
- The JVM interprets or compiles the bytecode into native machine code specific to the underlying system, allowing Java programs to run on any platform with a compatible JVM.
This design eliminates the need to recompile Java programs for different operating systems, making Java platform-independent.
4. What is the Diamond Problem, and How Does Java Solve It?
The Diamond Problem arises in object-oriented programming languages that support multiple inheritance. It occurs when a class inherits from two classes that share a common base class, leading to ambiguity about which implementation to use from the base class.
Example of the Diamond Problem:
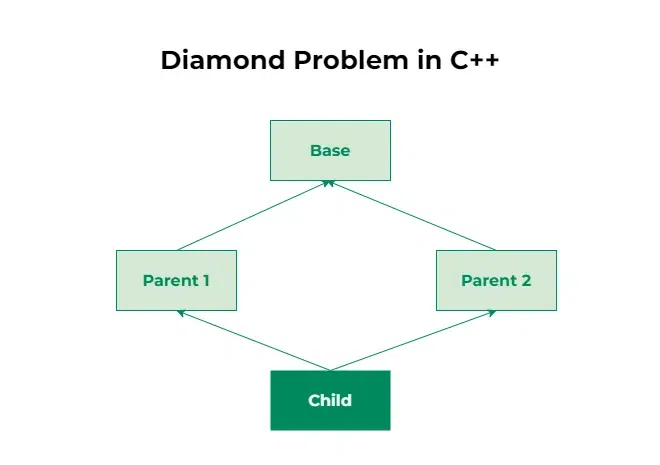
- Class
D
inherits from both B
and C
, which in turn inherit from A
.
- If both
B
and C
override a method from A
, and D
calls that method, it becomes unclear which version to use—B
’s or C
’s.
How Java Solves It:
- Java does not support multiple inheritance with classes.
- A class can only inherit from a single class in Java, eliminating the Diamond Problem.
- Instead, Java uses interfaces to achieve multiple inheritance-like behavior:
- Interfaces allow a class to inherit method signatures from multiple sources.
- If there are conflicts in default method implementations (introduced in Java 8), the programmer must resolve the ambiguity explicitly by overriding the conflicting method in the derived class.
Example in Java:
interface A {
default void display() {
System.out.println("Display from A");
}
}
interface B {
default void display() {
System.out.println("Display from B");
}
}
class C implements A, B {
@Override
public void display() {
// Explicitly choosing which implementation to use or providing a new one
A.super.display(); // Calls A's display method
}
}
public class DiamondProblemExample {
public static void main(String[] args) {
C obj = new C();
obj.display(); // Resolves ambiguity
}
}
By preventing multiple inheritance in classes and resolving ambiguities in interfaces, Java effectively avoids the Diamond Problem.